Core Java Interview Questions and Answers (2024)
Ace your 2024 Core Java interviews with this guide. Get essential questions, expert answers, and tips to succeed. Ideal for ambitious developers.
Cracking a Java interview requires a strong foundation in core Java concepts. This guide equips you with precisely that, offering up-to-date and comprehensive explanations alongside essential interview questions for 2024. Whether you're a seasoned developer or a budding programmer, this resource will help you refresh your knowledge, tackle common challenges, and showcase your expertise to potential employers.
For those looking to leverage their core Java skills in a new career opportunity, Weekday offers a unique platform that connects talented engineers with top tech companies.
1. What is Core Java and its significance in software development?
Core Java refers to the subset of Java SE (Standard Edition), which includes the fundamentals of the Java programming language. It is used for building general-purpose applications and serves as the foundation for learning advanced technologies and frameworks in Java. Core Java covers essential topics like syntax, data types, operators, control structures, classes, objects, inheritance, exceptions, and I/O operations.
The significance of Core Java in software development lies in its platform independence, robustness, and object-oriented nature, making it suitable for a wide range of applications, from desktop applications to server-side solutions. The principles learned in Core Java provide a solid foundation for understanding more complex frameworks and technologies used in Java EE (Enterprise Edition) for web and enterprise-level applications.
2. How do JDK, JRE, and JVM differ from each other?
The JDK (Java Development Kit), JRE (Java Runtime Environment), and JVM (Java Virtual Machine) are core components of Java but serve different purposes:
- JDK: This is the complete Java software development kit that includes JRE and development tools like compilers and debuggers, necessary for developing Java applications.
- JRE: This includes the JVM and library classes necessary for running Java applications. It does not include development tools and is meant for end-users who run Java applications but do not develop them.
JVM: This is the engine that provides the runtime environment in which Java bytecode can be executed. It is platform-dependent and is part of JRE. JVM is responsible for converting bytecode into machine-specific code.
3. What are the fundamental Object-Oriented Programming principles in Java?
Java supports the following fundamental Object-Oriented Programming (OOP) principles:
- Encapsulation: Encapsulating the data (attributes) and code (methods) together as a single unit or class. It also hides data access from the outside world and is only accessible through any member function of its class.
- Inheritance: This allows a new class to inherit the properties and methods of an existing class. In Java, inheritance is supported by using the extends keyword.
- Polymorphism: This allows one interface to be used for a general class of actions. The specific action is determined by the exact nature of the situation. In Java, polymorphism is mainly divided into two types: method overloading and method overriding.
- Abstraction: This is the concept of hiding the complex reality while exposing only the necessary parts. It can be achieved in Java using abstract classes and interfaces.
Enhance your OOP skills with practical experience by exploring career opportunities on Weekday, where innovative companies look for talented Java developers who excel in encapsulation, inheritance, polymorphism, and abstraction.
4. What are data types, variables, and operators in Java?
- Data types: In Java, data types specify the size and type of values that can be stored in a variable. There are two types: primitive (such as int, double, char) and non-primitive or reference types (such as String, arrays, and classes).
- Variables: Variables are containers for storing data values. In Java, each variable must be declared with a data type that defines the type and the operations that can be performed on the variable.
- Operators: Operators are special symbols or keywords that are used to perform specific operations on one, two, or three operands. Java operators include arithmetic, relational, logical, assignment, and more.
5. How are control flow statements like if-else, switch, for, while, and do-while used in Java?
Control flow statements regulate the flow of execution depending on the conditions at runtime:
- if-else: Used to execute a block code when a condition is true. If the condition is false, the code in the else block is executed.
- switch: A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each case.
- for: Used to execute a block of code a set number of times. This loop includes the initialization, condition, and increment/decrement in one line, making it easy to understand and manage.
- while: Executes a block of code as long as the specified condition is true.
- do-while: Similar to the while loop, but it guarantees to execute the block of code at least once before checking the condition.
These structures are fundamental to directing the flow of any Java program, allowing for complex algorithms to be implemented effectively.
6. What are classes and objects in Java, and how are they different?
Classes and objects are fundamental concepts of object-oriented programming in Java. A class is a blueprint or prototype from which objects are created. It defines a datatype by bundling data and methods that work on the data into one single unit. For example, you might have a class Car that includes attributes like color, brand, and horsepower, along with methods like drive and brake.
An object is an instance of a class. When you create an object, you are creating an instance with a defined structure as declared in the class, but with its own individual state. For example, if a Car is a class, then a specific car like a red Tesla Model S could be an object of the Car class with its attributes set to particular values.
The key difference is that a class is a template for creating objects, and an object is an instance of a class with actual values.
7. What are the types and uses of inheritance in Java?
Inheritance in Java is a mechanism where a new class is derived from an existing class. The class from which the subclass is derived is called a superclass, and the class that is derived is called a subclass. Java supports several types of inheritance:
- Single Inheritance: Where a class extends another class, inheriting all its properties and methods. For example, if class Bicycle extends class Vehicle, then Bicycle inherits everything from Vehicle.
- Multilevel Inheritance: This involves a chain of inheritance. For example, if class Car extends Bicycle, and Bicycle extends Vehicle, then Car inherits attributes and methods of both Bicycle and Vehicle.
- Hierarchical Inheritance: Where one superclass has multiple subclasses. For example, a Vehicle class might be extended by both Bicycle and Car classes.
Java does not support multiple inheritance with classes (to avoid "Diamond Problem"), but it can be achieved with interfaces.
8. Explain compile-time and run-time polymorphism in Java.
Polymorphism in Java comes in two main types: compile-time and run-time polymorphism.
- Compile-time Polymorphism (also known as method overloading) occurs when two or more methods in the same class have the same name but different parameters (different number of parameters, different types of parameters, or both). The method call is resolved at compile time.
- Run-time Polymorphism (also known as method overriding) occurs when a subclass has a method with the same name, return type, and parameters as a method in its superclass. The method to be called is determined at runtime, based on the object type through which it is called, not the reference type.
9. Can you provide real-world examples of encapsulation and abstraction in Java?
Encapsulation is the technique of making the fields in a class private and providing access via public methods. A real-world example could be a BankAccount class where the balance is kept private to prevent unauthorized modifications. Public methods like deposit() and withdraw() are provided to manage the balance securely.
Abstraction means hiding the complex implementation details of a system and exposing only the necessary parts of it. For example, consider a smartphone where you interact with a simple user interface to send messages, but the underlying technology and complex processes (like signal processing, and data encoding) are hidden.
10. When should interfaces and abstract classes be used in Java?
Interfaces and abstract classes are used to achieve abstraction in Java, but they serve different purposes and have different rules:
- Interfaces are used when you want to specify that certain classes should comply with a certain contract (methods that must be implemented) without specifying how these methods should be implemented. Use interfaces when various implementations only share method signatures, not code.
- Abstract classes are used when classes share a common structure (code) and behavior (methods), with some core methods that should be implemented differently. You should use an abstract class when multiple classes have common methods or fields, and you want to provide a partially complete implementation.
Both are tools for abstraction, but the choice depends on the specific design and what you're trying to accomplish.
11. How do String, StringBuilder, and StringBuffer differ in Java?
String, StringBuilder, and StringBuffer are classes in Java used for working with text data, but they have key differences:
- String: Objects of this class are immutable, which means once a String object is created, its value cannot be changed. Any operation that alters the String actually creates a new String object.
- StringBuilder: This class provides an alternative to String when you need a mutable sequence of characters. StringBuilder is not synchronized, which means it's not thread-safe but it's faster and should be used when thread safety is not a concern.
- StringBuffer: Similar to StringBuilder, StringBuffer also allows a mutable sequence of characters. However, it is thread-safe because all of its public methods are synchronized. This makes it a good choice if you need to modify strings from multiple threads but is less efficient in single-threaded scenarios.
12. How do you work with single and multidimensional arrays in Java?
In Java, arrays are containers that hold a fixed number of elements of a single type. The length of an array is established when the array is created and cannot be changed.
- Single-dimensional arrays: You declare, instantiate, and initialize them as follows:

Multidimensional arrays: Essentially arrays of arrays. The most common type is the two-dimensional array:

- You can iterate through either type of array using loops, such as for-loop or enhanced for-loop.
13. What is the overview of the Collections Framework in Java?
The Java Collections Framework is a set of classes and interfaces that implement commonly reusable collection data structures. It includes classes like ArrayList, HashSet, and TreeMap, and interfaces like List, Set, and Map. This framework provides several benefits:
- Reduces programming effort by providing data structures and algorithms so you don't have to write them yourself.
- Increases quality because each collection is well-designed, well-implemented, and widely used.
- Supports interoperability between different APIs that rely on collection interfaces.
14. How are lists, sets, and maps used in Java, and what are their differences?
- Lists (implementations include ArrayList, and LinkedList): Ordered collections that can contain duplicate elements. They allow positional access and insertion of elements.
- Sets (implementations include HashSet, LinkedHashSet, TreeSet): Collections that do not allow duplicates and are typically used to model the mathematical set abstraction. They are primarily used for membership operations like add, remove, and contain.
- Maps (implementations include HashMap, TreeMap, LinkedHashMap): Objects that map keys to values. A map cannot contain duplicate keys, and each key can map to at most one value. Maps are used when you need efficient retrieval of data based on a key.
15. What are generics in Java, and what are their benefits and basic usage?
Generics provide a way to introduce type parameters to classes, interfaces, and methods, much like templates in C++. The use of generics provides the following benefits:
- Stronger type checks at compile time. A Java compiler applies strong type checking to generic code and issues errors if the code violates type safety.
- Elimination of casts. If you use generics, you do not need to cast the object.
- Enabling programmers to implement generic algorithms. By using generics, programmers can implement generic algorithms that work on collections of different types, can be customized, and are type safe.
Basic usage of generics involves declaring a generic type with a type parameter:

16. How is exception handling done in Java using try, catch, finally, throw, and throws?
In Java, exception handling is a critical mechanism that helps in managing runtime errors, ensuring the program continues to run without crashing.
- try block: Code that might throw an exception is placed inside a try block. If an exception occurs, it is thrown.
- catch block: This block catches the exception thrown by the try block. You can have multiple catch blocks to handle different types of exceptions.
- finally block: This block is optional and executed after a try/catch block has completed but before the code following the try/catch. It executes regardless of whether an exception was thrown or caught.
- throw: This keyword is used to explicitly throw an exception, either a newly instantiated one or an exception that was caught.
- throws: Used in a method's declaration to indicate that this method might throw one or more exceptions. The calling method is responsible for handling these exceptions.
Example of using these keywords:

17. Explain the difference between checked and unchecked exceptions in Java.
In Java, exceptions under the Throwable class are categorized into two types: checked exceptions and unchecked exceptions.
- Checked Exceptions: These are exceptions that must be either handled with a try-catch block or declared to be thrown in the method's signature using the throws keyword. They are called 'checked' because the compiler checks at compile-time to ensure that these exceptions are appropriately handled or declared. This category includes exceptions like IOException, SQLException, etc. The intent is to make the developer aware of possible exceptions, encouraging a proactive handling strategy.
- Unchecked Exceptions: These exceptions are not checked at compile time, meaning the compiler does not require methods to catch or declare them. Unchecked exceptions are derived from the RuntimeException class and include exceptions like NullPointerException, ArithmeticException, etc. They generally result from programming errors such as faulty logic, incorrect API usage, or other runtime mishaps that could have been prevented by the programmer.
18. How do FileReader, FileWriter, FileInputStream, and FileOutputStream handle file operations in Java?
Java provides various classes to handle file operations, with FileReader, FileWriter, FileInputStream, and FileOutputStream being some of the primary ones used for reading and writing files.
- FileReader and FileWriter: These classes are part of the java.io package and are used for reading and writing text files. FileReader reads files character-by-character, making it suitable for text files. FileWriter is used to write characters to a file. Both classes handle characters, which makes them suitable for text data.
- FileInputStream and FileOutputStream: These are also part of the java.io package but are used for reading and writing binary files. FileInputStream reads bytes from a file, making it suitable for any type of file, including images, video, or binary data. FileOutputStream writes bytes to a file. These are byte-oriented classes and do not interpret the bytes, making them ideal for raw binary data.
19. What are the basics of multithreading and concurrency in Java, including thread lifecycle and synchronization?
Multithreading and concurrency are foundational concepts in Java that allow multiple threads to run concurrently, leading to better utilization of CPU resources.
Basics of Multithreading: In Java, a thread is a lightweight process, and multithreading is the capability of a CPU to execute multiple threads concurrently. Java supports multithreading through its java.lang.Thread class and java.util.concurrent package. Each thread runs in a separate call stack.
Thread Lifecycle: The lifecycle of a thread in Java includes several states:
- New: The thread is created but not yet started.
- Runnable: The thread is executing in the Java virtual machine but may be waiting to be selected for execution by the thread scheduler.
- Blocked: The thread is blocked waiting to acquire a monitor lock.
- Waiting: The thread is waiting indefinitely for another thread to perform a specific action.
- Timed Waiting: The thread is waiting for another thread to perform an action up to a specified waiting time.
- Terminated: The thread has exited its run method and is complete.
- Synchronization: Synchronization in Java is used to control the access of multiple threads to shared resources. Without synchronization, it is possible for multiple threads to interfere with each other, leading to inconsistent data. Synchronization can be achieved in Java using the synchronized keyword, which locks an object for exclusive use by a thread. Another way to handle synchronization is through new concurrency utilities like the classes found in the java.util.concurrent package, which provide higher-level constructs such as locks, blocking queues, and semaphores.
20. What are the Java 8 features such as lambda expressions, Stream API, and default methods in interfaces?
Java 8 introduced several major features that significantly enhanced the language's capabilities, particularly in terms of functional programming and code conciseness:
- Lambda Expressions: These are anonymous functions that provide a clear and concise way to represent one method interface using an expression. Lambda expressions are used primarily to define the implementation of a method provided by a functional interface directly in-line and can be treated as a method argument or code data.
- Stream API: Introduced in Java 8, the Stream API is used to process collections of objects in a functional manner. The Stream API provides a high-level abstraction for operations on collections, including sequential and parallel aggregate operations. Common operations include map, filter, reduce, find, match, sort, and collect.
- Default Methods in Interfaces: Java 8 allows interfaces to have default methods with implementation (non-abstract methods). This feature enables developers to add new methods to interfaces without breaking the existing implementation of these interfaces. It helps in enhancing the interfaces without having the need to modify the subclasses that implement these interfaces.
21. How do you connect to databases using JDBC in Java?
JDBC (Java Database Connectivity) provides a standard API for connecting databases in Java, allowing for database interactions through establishing connections, executing SQL queries, and managing result sets. Here’s a simplified process:
- Load the Driver: Identify the JDBC driver you need for the database you are using (like MySQL, PostgreSQL, Oracle) and load it using Class.forName("com.mysql.jdbc.Driver").
- Establish a Connection: Use DriverManager.getConnection() with the appropriate database URL, username, and password to establish a connection to the database.
- Create a Statement: Create a Statement or PreparedStatement object using the connection object. This object is used to execute queries.
- Execute Queries: Use the statement object to execute queries with executeQuery() for retrieving data or executeUpdate() for updating data.
- Process the Results: Process the results returned in a ResultSet for queries fetching data.
- Close Connections: Finally, close all connections, statements, and result sets to free up resources.
22. What are annotations and their use in Java?
Annotations are a form of metadata that provide data about a program but are not part of the program itself. Introduced in Java 5, annotations are used to provide additional information that can be used by the Java compiler or runtime environment. Common uses include:
- Compiler Instructions: Direct the Java compiler to suppress specific warnings.
- Compile-Time and Deployment-Time Processing: Software tools can process annotation information to generate code, XML files, etc.
- Runtime Processing: Some annotations are available to be examined at runtime.
Examples include @Override, @Deprecated, @SuppressWarnings, @FunctionalInterface, and various annotations in frameworks like Spring and Hibernate such as @Entity, @Autowired, etc.
23. Explain Java Memory Management, including Garbage Collection and Memory Leaks.
Java Memory Management involves the allocation and deallocation of objects to manage the heap space efficiently, where the heap is the area of memory used for dynamic memory allocation.
- Garbage Collection (GC): Java uses garbage collection to automatically manage memory. The garbage collector removes objects that are no longer being used by a program, freeing up memory automatically. Different types of garbage collectors include Serial GC, Parallel GC, CMS, G1 GC, each with its own method of managing memory.
- Memory Leaks: These occur when objects that are no longer needed are still referenced by a program, thus preventing the garbage collector from deallocating those objects. Over time, memory leaks can cause a program to consume increasing amounts of memory, potentially leading to performance issues or application crashes.
24. How are code organization and modularity achieved using SOLID principles in Java?
SOLID principles are a set of design principles for object-oriented programming and design, intended to make software designs more understandable, flexible, and maintainable. In Java, these principles help in achieving better code organization and modularity:
- Single Responsibility Principle: Every class should have a single responsibility and thus only a single reason to change.
- Open/Closed Principle: Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions.
Adhering to these principles helps in developing systems that are more modular, where individual components can be developed, tested, modified, and replaced more easily and independently.
25. Can you explain Singleton, Factory, Observer, and other design patterns used in Java?
Design patterns are established solutions to common software design problems. Here are explanations for a few fundamental patterns:
- Singleton Pattern: This pattern ensures that a class has only one instance and provides a global point of access to it. The Singleton class seals its constructor to prevent the creation of the object through new and provides a static method that returns the sole instance. The Singleton pattern is useful for managing resources such as database connections or settings.
- Factory Pattern: Factory pattern is a creational pattern used to create objects. Instead of directly invoking the constructor, the object creation is handled by a factory method, which makes the program more flexible by delegating the instantiation of objects to child classes. The Factory pattern is commonly used where a class cannot anticipate the type of objects it needs to create beforehand.
- Observer Pattern: This behavioral pattern is used when a change to one object (subject) requires changing others (observers), and you want to maintain consistency between related objects without making classes tightly coupled. The subject maintains a list of observers and notifies them of any changes, usually by calling one of their methods.
- Other Common Patterns:
- Decorator Pattern: Allows for adding new functionality to an existing object without altering its structure. This is achieved by wrapping them with a new class.
- Strategy Pattern: Enables selecting the algorithm at runtime. Instead of implementing a single algorithm directly, code receives run-time instructions as to which in a family of algorithms to use.
26. What are the unit testing frameworks JUnit and TestNG in Java?
JUnit and TestNG are popular frameworks for performing unit tests in Java:
- JUnit: This is one of the most popular unit-testing libraries for Java. It is an open-source framework designed to write and run tests in the Java programming language. JUnit is known for its simplicity and ease of use, especially for testing small units of code.
- TestNG (Test Next Generation): Inspired by JUnit but introduced with new functionalities making it more powerful and easier to use, TestNG is used for a wide range of testing needs, from unit testing to integration testing. It supports more complex tests with features like annotations, grouping, sequencing, and parameterization of tests.
Both frameworks allow you to write code faster, which increases the quality. They provide annotations to identify test methods, assertions to test expected results, and test runners for running tests.
27. How do you debug and optimize Java code effectively?
Debugging and optimizing Java code are crucial for improving application performance and maintainability:
- Debugging:
- Use of IDE Features: Modern IDEs (Integrated Development Environments) like IntelliJ IDEA and Eclipse provide powerful debugging tools, including breakpoints, step execution, variable inspection, and call stack analysis.
- Logging: Implementing logging frameworks like Log4J or SLF4J can help track down problems by recording runtime information, which can be vital for understanding the behavior of a system.
- Optimization:
- Profiling Tools: Tools like VisualVM, JProfiler, or YourKit provide insights into memory usage, CPU usage, and thread behavior, helping identify bottlenecks.
- Code Optimization: Techniques include optimizing algorithms, reducing memory consumption, and minimizing synchronization to enhance performance.
- Best Practices: Following best practices such as using appropriate data structures, avoiding premature optimization, and understanding JVM options can significantly affect performance.
By effectively utilizing these strategies, developers can enhance the reliability and performance of Java applications, making them more robust and efficient.
Tips for Preparing for a Core Java Interview
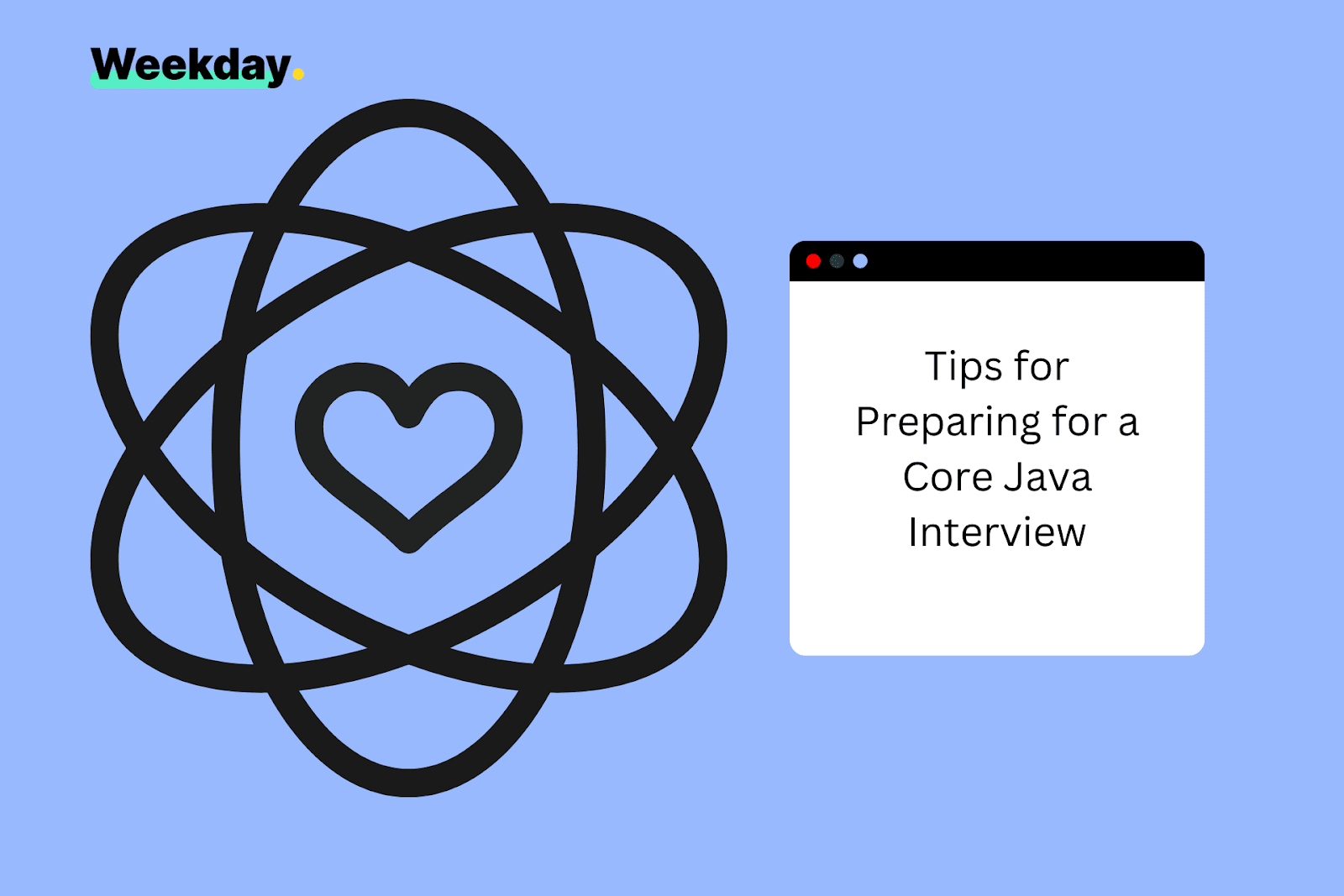
Preparing for a Core Java interview requires a combination of solid understanding of Java fundamentals, hands-on practice, and familiarity with commonly asked questions. Below, I’ve outlined several tips and resources to help you get ready for a Core Java interview in 2024.
Understanding the Format of Core Java Interviews in 2024
Core Java interviews in 2024 typically follow a multi-stage format, combining technical assessments with behavioral evaluations:
- Technical Screening: Often a phone or video call focusing on basic Java concepts, such as OOP principles, exception handling, collections, and threading.
- Coding Challenge: This could be a take-home assignment or an online coding test conducted on platforms like HackerRank or Codility, testing your problem-solving abilities and coding skills.
- Technical Deep Dive: In-depth discussions about advanced Java topics, design patterns, system design, and possibly specific frameworks or libraries depending on the job role.
- Behavioral Interview: Evaluate your problem-solving approach, teamwork, leadership skills, and how you handle pressure and failures.
- Culture Fit & HR Round: Typically the final round to assess how well you align with the company’s culture and values.
Recommended Books and Resources for Preparation
- Books:
- Online Documentation and Tutorials:
Online Platforms for Mock Interviews and Practice
- LeetCode: Offers a vast range of coding problems and a dedicated section for mock interviews. Its problems are categorized by difficulty and topic.
- HackerRank: Provides a good collection of Java questions, which helps in practicing specific concepts in Java.
- Interviewing.io: You can practice mock interviews anonymously with engineers from top tech companies, which helps simulate real interview environments.
- Pramp: A free platform that allows you to practice technical interviews with peers. Pramp provides a half-hour mock interview platform where you can both interview others and be interviewed.
As you prepare for your next Java interview, remember that Weekday is your go-to platform to connect with leading tech companies looking for skilled Java developers.
Conclusion
As you prepare for Core Java interviews in 2024, having a strong grasp of the fundamentals and understanding advanced concepts is crucial. This guide has equipped you with essential information, from interview formats to key Java features and effective preparation strategies. For further enhancement of your Java skills and to connect with top tech opportunities, consider visiting Weekday, where you can find vetted engineering roles and expand your professional network. Dive deeper into your Java preparation and explore exciting career opportunities with Weekday.